Exploring the Power of React Components: Building Dynamic Web Applications
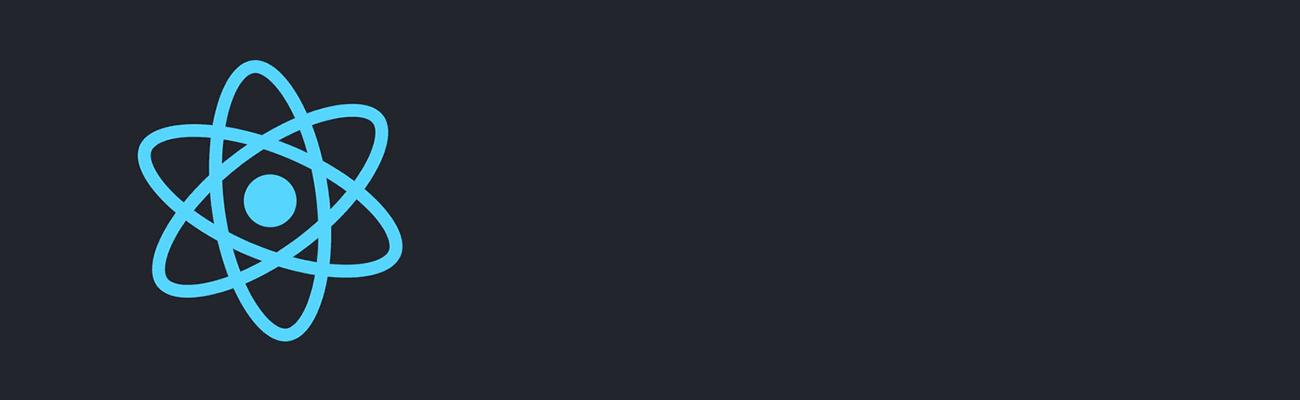
Why React Components Matter
Welcome to the fascinating world of React components. We explore the power behind React web components and how they make web development easier and more efficient. This article will provide you with information that can assist you in defining and organizing React components while building your next project. You will also learn different techniques for creating components and sharing data between them. Moreover, you will gain knowledge about various testing methods, tools, and libraries.
React components are like building blocks that users can join together to create complex and interactive user interfaces. Each component has its own functionality that you can use and reuse throughout your application. This approach saves time and effort.
Once you understand this, you’ll be able to have a conversation with a React developer about some of the intricacies that are possible in your application. We will cover the basics of React components, different types of React components, their reusability, lifecycle, styling, and testing. These factors collectively add to the power and usability of React components.
Understanding React Components
A React component is like a small, reusable bit of code. Think of it as a puzzle piece that you can use to build a great website. Each component represents a specific part of your app, like a button, a menu, or a form. By breaking your app into these smaller components, you can keep your code organized and make it easier to work with later on. It’s a smart way to build websites that look good and work well.
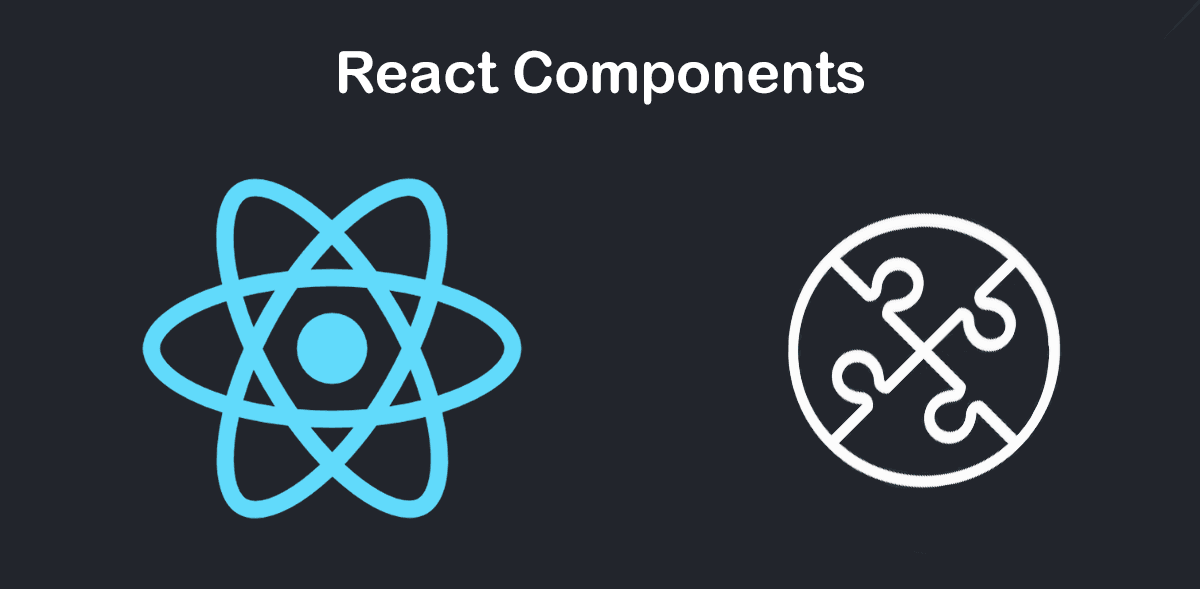
If you’re looking to learn the very basics of React you can start here first. If not, let’s jump right in. The flexibility of React components enables developers to use them for building UI components in various ways. Developers can use these components over and over again in different projects. This greatly simplifies and speeds up web development, while ensuring a consistent look and functionality across your website or application without having to reinvent the wheel every time.
Types of React Components
The React library offers two types of components: functional components. and class components.
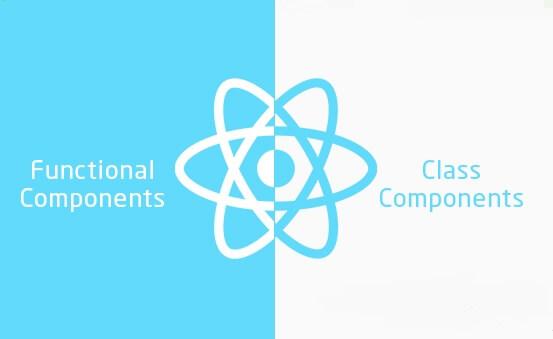
React Functional Components
React Functional components, also referred to as stateless presentational components, are the fundamental and recommended React component type. They are simple JavaScript functions that accept props (properties) as arguments and produce JSX (JavaScript XML) as their result. Developers often use functional components to display information. Their main purpose is to streamline the process of rendering the user interface. By design, they prioritize simplicity and specialize in generating UI elements based on the provided props.
React Class Components
React class components are sophisticated components designed for handling complex logic. Users often refer to these components as “stateful” or “container” components because they have the ability to manage “state”, a way to store and manage data that can change over time. In React, we can create class-based components using JavaScript ES6 classes. These class components provide a seamless way to communicate with other components, allowing for efficient data sharing between them. They play a crucial role in React applications, particularly when a component needs to manage state, handle side effects, or interact with lifecycle events.
Reusability and Composition
In React, reusability is a fundamental concept that allows us to use components multiple times without rewriting code. It’s like a template that you can use whenever needed. For example, you can create a Button Component once and reuse it throughout the website, saving time and effort. Developers can customize these components with unique properties, allowing for different appearances and behaviors. An advantage is that changes to the component can be made in one place, such as updating its style, and all instances will automatically reflect the change. It makes maintenance much easier!
Another key concept in React is composition, which involves combining multiple components to create larger components or more complex UI. The true advantage of component composition lies in its ability to enhance reusability and maintainability. It also makes your code more organized and easier to understand. When making changes or adding new features, developers can focus on specific components without worrying about the rest of the codebase.
Props and State
In the context of software development, you will often notice the terms “props” and “state.” Props and state are both plain JavaScript objects. Let’s take a closer look at the Props and State:
Props
In React, props serve as objects that store attribute values for tags. The term “props” is an abbreviation for “properties,” and their functionality closely resembles HTML attributes. Essentially, props act as read-only components, meaning they cannot be altered within the component itself. In React, developers can use props to sent data between components, much like passing arguments in a function. To illustrate, suppose, we have a “Button” component, we can pass a prop called “text” to specify the desired text to be shown on the button.
State
The state functions as the internal memory of a component that is used to contain data or information about the component. Unlike props, the state is mutable, meaning it can be changed within the component. You can utilize the state is when you want your component to keep track of something that can change over time. To illustrate, consider a “Counter” component where the state can be employed to store and dynamically update the ongoing count value.
Lifecycle Methods and Hooks
Component Lifecycle methods
You can think of React lifecycle methods as the series of events that happen during the existence of a React component. These methods allow developers to control and perform specific actions during various phases of a component’s lifecycle. The lifecycle methods fall into three main phases:
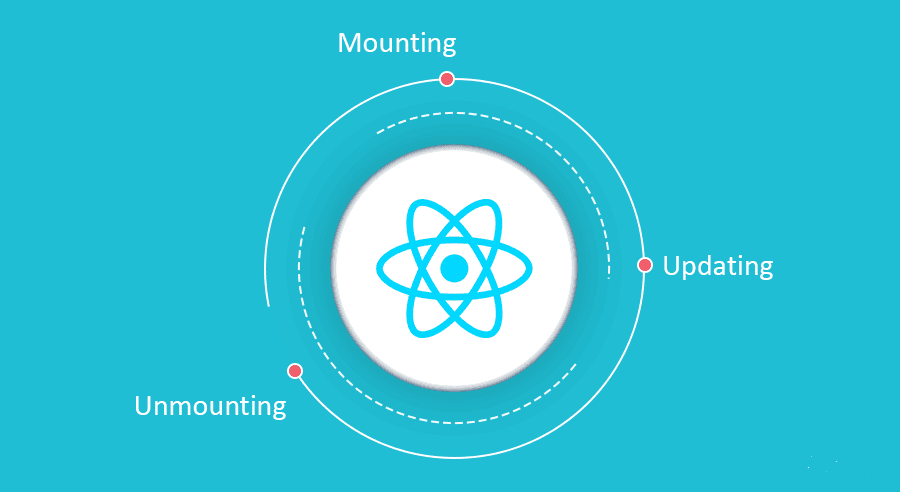
Mounting:
Your app calls these methods when an instance of a component is being created and added to the DOM. The mounting phase has three main lifecycle methods that are:
constructor()
: The constructor() method is called when the component is first created.
render()
: The render() method is responsible for generating the component’s virtual DOM representation based on its current props and state.
componentDidMount()
: The componentDidMount() method is called once the component has been mounted into the DOM.
Updating:
The app calls these methods when a component is being re-rendered due to changes in props or state. The updating phase uses these methods:
componentDidUpdate(prevProps, prevState)
: Invoked after the component has been updated and re-rendered.
shouldComponentUpdate(nextProps, nextState)
: Determines whether the component should be re-rendered or not.
Unmounting:
Your application calls this method when a component is being removed from the DOM. The following method is involved during the unmounting phase.
componentWillUnmount()
: Invoked just before the component is unmounted and destroyed.
Concept of React Hooks, their role, and examples
React Hooks are basically simple JavaScript functions which are reusable blocks of code that performs a specific task and can be called and executed multiple times throughout a program. React Hooks allow us to isolate the reusable part of a functional component. They provide a simpler and more concise way to manage state and side effects in functional components in a more organized way, bettering code readability and reusability.
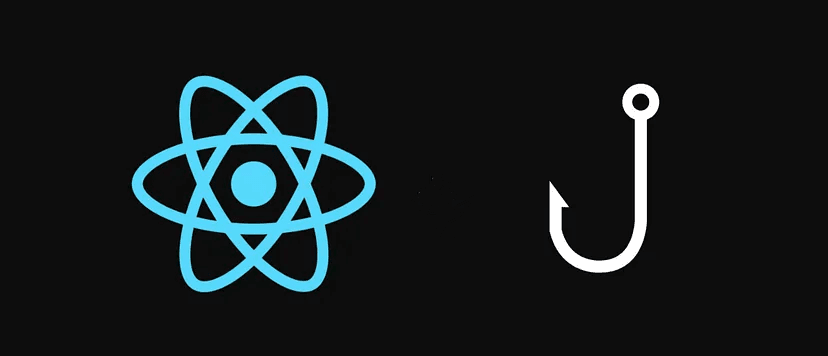
React provides several built-in hooks that we can use to enhance the functionality and maintainability of components. Here are some React hooks that developers commonly use:
useState: Manages component state with current value and update function.
useEffect: Handles side effects after component rendering, including cleanup.
useContext: Accesses the current value of a context for data sharing between components.
useReducer: Alternative to useState for advanced state management.
useCallback: Returns memoized callback function to prevent unnecessary re-renders.
useMemo: Returns memoized value for performance optimization.
useRef: Provides reference objects with .current property for accessing child components or storing mutable values.
useLayoutEffect: Handles side effects synchronously after DOM mutations.
useDebugValue: Displays label in React DevTools for custom hooks, aiding debugging.
Styling React Components
When we talk about styling in React, we’re referring to how the different parts of your React components or elements look on the screen. You can change things like the color, size, font, and layout of your components to make them look attractive and fit the design of your application. It’s all about making your React app look good and appealing to users.
Different methods for styling React components
There are several ways you can style React components, and the choice often depends on personal taste, project needs, and the tools or libraries you are using. Here are some common methods for styling React components:
Inline styles:
You can define styles directly within the component’s JSX using the style attribute. Inline styles are defined as JavaScript objects, where keys represent the CSS properties and values represent their related values. For example:
// JSX const MyComponent = () => { const styles = { color: "red", fontSize: "18px", fontWeight: "bold" }; return <div style={styles}>Hello, World!</div>; };
Pros | Cons |
Easy to use and understand. Inline CSS allows applying style rules to specific elements. Allows dynamic styling by using JavaScript variables or expressions. | Can be cumbersome and clutter the JSX code for complex styles. Limited reusability, as users must define styles within each component. Difficult to manage and update styles across multiple components. |
CSS files:
You can create separate CSS files and import them into your React components. This allows you to separate the styling concerns from your component logic. To use CSS classes defined in your files, you can assign them to the className attribute of your JSX elements. For example:
// JSX import "./MyComponent.css"; const MyComponent = () => { return <div className="my-component">Hello, World!</div>; };/* MyComponent.css */ .my-component { color: red; font-size: 18px; font-weight: bold; }
Pros | Cons |
Popular and widely-used approach, with strong community and tooling. It is easier to make changes to the style when needed. Allows reusability of styles across multiple components. | The global scope can lead to style conflicts if class names are not unique. Requires managing and importing CSS files into components. May require extra build configurations or loaders, depending on the tooling used. |
CSS Modules:
CSS Modules provide a way to locally scope CSS class names, avoiding conflicts between different components. When using CSS Modules, you import the CSS file and reference the generated unique class names in your component. For example:
// JSX import styles from "./MyComponent.module.css"; const MyComponent = () => { return <div className={styles.myComponent}>Hello, World!</div>; };/* MyComponent.module.css */ .myComponent { color: red; font-size: 18px; font-weight: bold; }
Pros | Cons |
Modular and reusable CSS No more styling conflicts Explicit dependencies Local scope | Mixing CSS Modules and global CSS classes is cumbersome. May require extra build tools. |
CSS-in-JS libraries:
CSS-in-JS libraries are tools that allow developers to write and manage CSS styles directly in JavaScript code. Instead of having separate CSS files, these libraries enable the creation of styles within the JavaScript components themselves. Here’s an example using styled-components:
// JSX import styled from "styled-components"; const MyComponent = styled.div` color: red; font-size: 18px; font-weight: bold; `; const App = () => { return <MyComponent>Hello, World!</MyComponent>; };
Pros | Cons |
Offers a seamless integration of styles with React components. Allows writing styles directly within the component code, improving readability and maintainability. Provides dynamic styling capabilities using props or theme variables. Automatically handles scoping and avoids global style conflicts. | Requires higher learning curve and familiarization with the library’s syntax. May introduce more build dependencies and increase bundle size. Can be less familiar to developers coming from traditional CSS approaches. |
Testing and Debugging React Components
Importance of testing components for ensuring their functionality
React Testing is all about verifying what was specified and what was delivered. It aims to ensure that the developed application meets the identified functional, performance, design, and implementation requirements outlined in the procurement specifications. As React applications grow in complexity, the importance of testing also increases.
The following points highlight the significance of React testing for developing an efficient software product:
The testing is significant since it detects bugs before the product is delivered to the client, which guarantees the quality and efficiency of the software.
Thoroughly tested software ensures reliability and high-performance software operation.
It enhances the software’s efficiency, reliability, and user-friendliness.
Popular testing libraries and frameworks for React
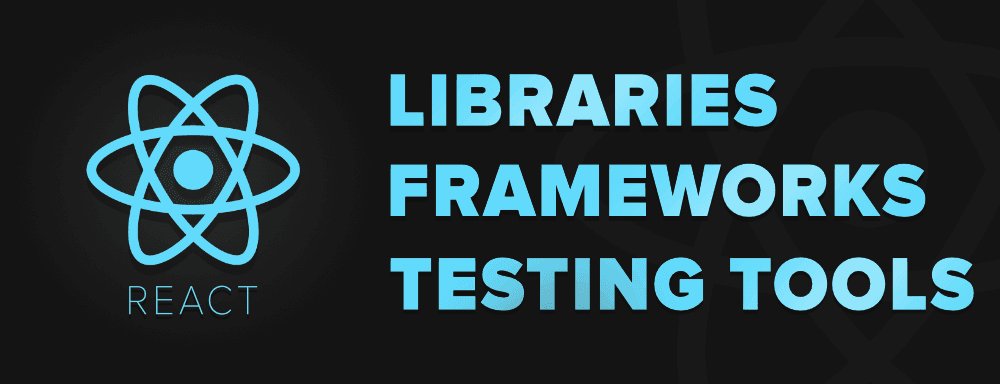
Testing the user interface of all the applications before they are released to the customer is a must. Developers can test web-based applications using a variety of methods. Many open-source developers have combined these testing methods into reusable libraries. There are several libraries available for testing React components in the React community. Some of the top react testing libraries and frameworks are as follows:
Jest
Jest is a widely used JavaScript testing framework that includes a test runner and assertion functions, making it beginner-friendly and efficient for testing code. It boasts high-performance execution and supports features such as snapshots, parallelization, and asynchronous method tests.
Mocha
Mocha is a popular test framework for JavaScript that offers developers complete control over code testing. It is compatible with Node.js and supports both front-end and back-end asynchronous testing. Mocha stands out in error tracking and users can use it with Enzyme and Chai for assertions and mocking in React-based web application testing.
Chai
Chai is a widely-used assertion and expectations library for JavaScript, compatible with both node and browser environments. Users can utilize Chai with any testing framework and developers commonly use it alongside Mocha for testing React applications. Chai provides multiple functionalities such as “expect,” “should,” and “assert” for declaring test expectations.
Cypress IO
Cypress IO is a fast end-to-end testing framework that removes the need for other testing frameworks. Developers can write and execute tests in real browsers or from the command line. It includes a control panel for monitoring test status and offers features like time travel with snapshots, screenshots, and videos, automatic waiting, network traffic control, built-in parallelization, and load balancing.
Enzyme
Enzyme is a testing utility created for the seamless testing of React components. Developers often use Enzyme alongside frameworks like Jest, Chai, or Mocha for testing React applications. Enzyme simplifies tasks such as rendering components, accessing and finding elements, simulating events, and testing component outputs.
React Components Shape Your Success
Considering all the points mentioned here, it is easy to understand the power of React components. React components have revolutionized the way developers build web applications. Their exceptional versatility, reusability, and maintainability allow developers to create amazing things by breaking down complex applications into reusable parts.
In this article, we learned about different types of React components, the concepts of props and state for dynamic data rendering and interactivity within components. We reviewed the lifecycle methods and hooks, noting how they provide control over component behavior. We also touched upon styling React components, emphasizing the flexibility and variety of styling options available. Finally, we recognized the significance of testing and debugging React components, highlighting popular testing libraries and frameworks.
Next Steps
We hope that this tutorial has provided you with a fundamental understanding of React components. Once you have grasped and applied these concepts, you will be able to fully utilize the potential of React components to create robust, scalable, and visually appealing web applications.If you found this article helpful, please share it and recommend it to others. I would also appreciate your ideas for future topics you’d like us to write about! Please reach out to us on Twitter with your interests and preferences. Thanks for your support and engagement!